Data processing using the apply Operator with OpenEO#
Apply Operator#
The apply operator employ a process on the datacube that calculates new pixel values for each pixel, based on n other pixels.
Let’s start again with the same sample data from the Sentinel-2 STAC Collection, applying the filters directly in the load_stac
call, to reduce the amount of data.
import openeo
from openeo.local import LocalConnection
local_conn = LocalConnection('')
url = "https://earth-search.aws.element84.com/v1/collections/sentinel-2-l2a"
spatial_extent = {"west": 11.259613, "east": 11.406212, "south": 46.461019, "north": 46.522237}
temporal_extent = ['2022-07-10T00:00:00Z','2022-07-13T00:00:00Z']
bands = ["red","green","blue"]
properties = {"eo:cloud_cover": dict(lt=50)}
datacube = local_conn.load_stac(url=url,
spatial_extent=spatial_extent,
temporal_extent = temporal_extent,
bands=bands,
properties=properties
)
datacube.execute()
Did not load machine learning processes due to missing dependencies: Install them like this: `pip install openeo-processes-dask[implementations, ml]`
/home/749c4c89-469c-4d4a-9ce4-9feffea0504a/.local/lib/python3.11/site-packages/stackstac/prepare.py:408: UserWarning: The argument 'infer_datetime_format' is deprecated and will be removed in a future version. A strict version of it is now the default, see https://pandas.pydata.org/pdeps/0004-consistent-to-datetime-parsing.html. You can safely remove this argument.
times = pd.to_datetime(
/home/749c4c89-469c-4d4a-9ce4-9feffea0504a/.local/lib/python3.11/site-packages/stackstac/prepare.py:408: UserWarning: The argument 'infer_datetime_format' is deprecated and will be removed in a future version. A strict version of it is now the default, see https://pandas.pydata.org/pdeps/0004-consistent-to-datetime-parsing.html. You can safely remove this argument.
times = pd.to_datetime(
<xarray.DataArray 'stackstac-b814a604da3396a4e43965fd3693671a' (time: 1, band: 3, y: 713, x: 1145)> dask.array<getitem, shape=(1, 3, 713, 1145), dtype=float64, chunksize=(1, 1, 606, 860), chunktype=numpy.ndarray> Coordinates: (12/54) * time (time) datetime64[ns] 2022-07-12... id (time) <U24 'S2B_32TPS_20220712_... * band (band) <U5 'red' 'green' 'blue' * x (x) float64 6.733e+05 ... 6.848e+05 * y (y) float64 5.155e+06 ... 5.148e+06 instruments <U3 'msi' ... ... proj:shape object {10980} gsd int64 10 common_name (band) <U5 'red' 'green' 'blue' center_wavelength (band) float64 0.665 0.56 0.49 full_width_half_max (band) float64 0.038 0.045 0.098 epsg int64 32632 Attributes: spec: RasterSpec(epsg=32632, bounds=(600000.0, 5090220.0, 709800.0... crs: epsg:32632 transform: | 10.00, 0.00, 600000.00|\n| 0.00,-10.00, 5200020.00|\n| 0.0... resolution: 10.0
Visualize the RGB bands of our sample dataset:
data = datacube.execute()
data[0].plot.imshow()
/home/749c4c89-469c-4d4a-9ce4-9feffea0504a/.local/lib/python3.11/site-packages/stackstac/prepare.py:408: UserWarning: The argument 'infer_datetime_format' is deprecated and will be removed in a future version. A strict version of it is now the default, see https://pandas.pydata.org/pdeps/0004-consistent-to-datetime-parsing.html. You can safely remove this argument.
times = pd.to_datetime(
Clipping input data to the valid range for imshow with RGB data ([0..1] for floats or [0..255] for integers).
<matplotlib.image.AxesImage at 0x7fee562919d0>
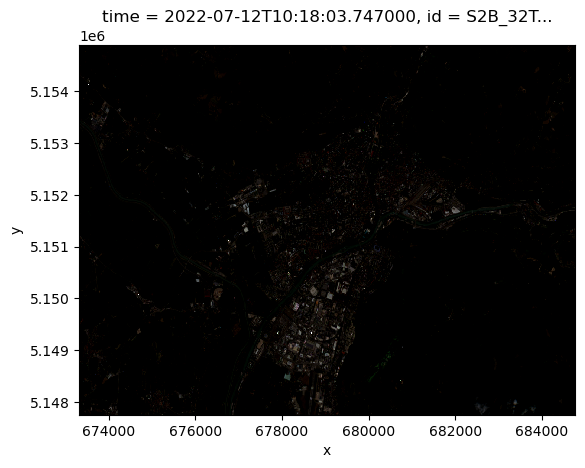
Apply an arithmetic formula#
We would like to improve the previous visualization, rescaling all the pixels between 0 and 1.
We can use apply
in combination with other math
processes.
from openeo.processes import linear_scale_range
input_min = -0.1
input_max = 0.2
output_min = 0
output_max = 1
def rescale(x):
return linear_scale_range(x,input_min,input_max,output_min,output_max)
scaled_data = datacube.apply(rescale)
scaled_data
Visualize the result and see how apply
scaled the data resulting in a more meaningful visualization:
scaled_data_xr = scaled_data.execute()
scaled_data_xr[0].plot.imshow()
/home/749c4c89-469c-4d4a-9ce4-9feffea0504a/.local/lib/python3.11/site-packages/stackstac/prepare.py:408: UserWarning: The argument 'infer_datetime_format' is deprecated and will be removed in a future version. A strict version of it is now the default, see https://pandas.pydata.org/pdeps/0004-consistent-to-datetime-parsing.html. You can safely remove this argument.
times = pd.to_datetime(
<matplotlib.image.AxesImage at 0x7fee57f68210>
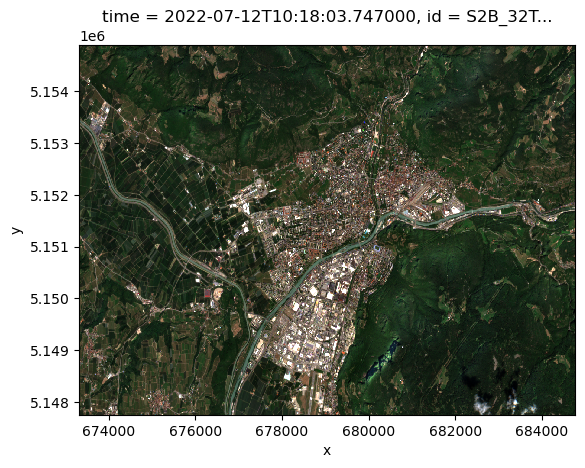