3.3 Data Sharing#
Science is much more impactful once it’s shared. Therefore, we are going to learn how to open up our scientific output from a cloud platform, so that is openly available - and has the chance to make the impact it should.
Reuse the workflow we have used before for creating the snow covered area
Select AOI,
Recreate process graph,
Download results for one time-step
A Snow Cover Area map in the COG format
A STAC metadata item that is provided with the result from openEO at CDSE
Adapt the STAC item
Upload the results and make them available openly via a STAC browser and web map
Libraries#
Start by creating the folders and data files needed to complete the exercise
!cp -r $DATA_PATH/33_results/ $HOME/
!cp $DATA_PATH/_33_cubes_utilities.py $HOME/
import json
import os
import subprocess
from datetime import datetime
import openeo
import numpy as np
import leafmap
import geopandas as gpd
import shapely
from shapely.geometry import Polygon
import matplotlib.pyplot as plt
from matplotlib.ticker import PercentFormatter
import rioxarray as rio
import xarray
from osgeo import gdal
from _33_cubes_utilities import (
calculate_sca,
visualize_bbox,
create_bounding_box,
extract_metadata_geometry,
extract_metadata_time
)
Login#
Connect to the copernicus dataspace ecosystem.
conn = openeo.connect('https://openeo.dataspace.copernicus.eu/')
Authenticate login
conn.authenticate_oidc()
Authenticated using refresh token.
<Connection to 'https://openeo.dataspace.copernicus.eu/openeo/1.2/' with OidcBearerAuth>
Check if the login worked
conn.describe_account()
Select an Area of Interest and Time Frame#
Start by selecting a center point of the area you would like to analyse from the map shown below. The starting extent is the full alps. Zoom in to an area and choose a region that has not been mapped yet. Make sure not to overlap too much with already mapped areas by having a look at the STAC Collection. It’s a community mapping project :) Create a 1 km bounding box around it. This will be the area you are calculating the snow covered area for.
Attention: Execute the cell below to show the map. Zoom to a location you want to analyze. Use the location symbol to select a point. A marker appears on the map. This is the center of your area of interest
m = leafmap.Map(center=(47.005, 11.507), zoom=7.5)
m
Attention: Now this cell will get the coordinates of the marker you have placed. This will create a 1 km bounding box around the chosen location. And visualize it in the map above. The marker moves to the center when you zoom in
feat = m.draw_features
geom = feat[0]['geometry']['coordinates']
# set distance of 1 km around bbox
distance_km = 1
# Create a bounding box around the point
bbox = create_bounding_box(geom[0], geom[1], distance_km)
visualize_bbox(m, bbox)
Now we’ll select the time frame. We’ll start with the winter months of 2023.
temporal_extent = ["2023-02-01", "2023-06-01"]
Reuse the process graph of the snow covered area data cube#
We’ve saved the python code that we had used to create the snow cover area data cube into a python function calculate_sca()
. It’s stored in cubes_utilities.py
. It creates a 4 dimensional data cube with the dimensions: x, y, time, bands.
As parameters we have exposed the bounding box and temporal extent. We will update them with the choices we have made above.
snow_map_4dcube = calculate_sca(conn, bbox, temporal_extent)
snow_map_4dcube
Reduce the time dimension#
We want to calculate the SCA for the winter period of a given year. Therefore, we need to reduce the values along the time dimension. We’ll use the process reduce_dimension()
with a median()
to accomplish this. We are directly continuing to build on our process graph that we have loaded above.
snow_map_3dcube = snow_map_4dcube.reduce_dimension(reducer="median", dimension="t")
snow_map_3dcube
Download result#
To finish our process graph we add the save_result()
process choosing the GTiff
format. It creates a COG out of the box with openEO on CDSE.
# create a batch job
snowmap_cog = snow_map_3dcube.save_result(format = "GTiff") #, options = {"overviews": "AUTO"})
We register the job as a batch job on the backend and start the processing. Depending on the traffic on the backend, this usually takes between 1 to 5 minutes.
job = snowmap_cog.create_job(title="snowmap_cog")
job.start_and_wait()
0:00:00 Job 'j-2401318f19a84122b62657ed798f0c9e': send 'start'
0:00:17 Job 'j-2401318f19a84122b62657ed798f0c9e': created (progress N/A)
0:00:22 Job 'j-2401318f19a84122b62657ed798f0c9e': created (progress N/A)
0:00:29 Job 'j-2401318f19a84122b62657ed798f0c9e': created (progress N/A)
0:00:37 Job 'j-2401318f19a84122b62657ed798f0c9e': created (progress N/A)
0:00:47 Job 'j-2401318f19a84122b62657ed798f0c9e': created (progress N/A)
0:01:00 Job 'j-2401318f19a84122b62657ed798f0c9e': created (progress N/A)
0:01:16 Job 'j-2401318f19a84122b62657ed798f0c9e': created (progress N/A)
0:01:36 Job 'j-2401318f19a84122b62657ed798f0c9e': created (progress N/A)
0:02:01 Job 'j-2401318f19a84122b62657ed798f0c9e': created (progress N/A)
0:02:31 Job 'j-2401318f19a84122b62657ed798f0c9e': created (progress N/A)
0:03:09 Job 'j-2401318f19a84122b62657ed798f0c9e': created (progress N/A)
0:03:56 Job 'j-2401318f19a84122b62657ed798f0c9e': created (progress N/A)
0:04:54 Job 'j-2401318f19a84122b62657ed798f0c9e': running (progress N/A)
0:05:54 Job 'j-2401318f19a84122b62657ed798f0c9e': finished (progress N/A)
Now let’s wait until the job is finished and then download the results.
if job.status() == "finished":
results = job.get_results()
results.download_files("33_results/")
Add statistics to the dataset via gdal, such as a summary of the values within the dataset and also some metadata, i.e. the legend (TIFFTAGS). And we reduce the datatype to the lowest possible datatype supported by COG uint8, since only have three values to represent (0, 1, 2). If you’re interested you can check what happened via !gdalinfo 33_results/openEO_uint8.tif
!gdal_translate -mo {TIFFTAG_IMAGEDESCRIPTION}=SnowCoveredArea_0=nosnow_1=snow_2-nodatavalue=cloud -ot Byte -of COG -a_nodata 2 -stats "33_results/openEO.tif" "33_results/openEO_uint8.tif"
Input file size is 145, 210
0...10...20...30...40...50...60...70...80...90...100 - done.
Load results#
Now we can open the COG and visualize it.
snowmap = rio.open_rasterio("33_results/openEO_uint8.tif", decode_coords="all")
snowmap
<xarray.DataArray (band: 1, y: 210, x: 145)> [30450 values with dtype=uint8] Coordinates: * band (band) int64 1 * x (x) float64 6.883e+05 6.884e+05 ... 6.898e+05 6.898e+05 * y (y) float64 5.169e+06 5.169e+06 ... 5.167e+06 5.167e+06 spatial_ref int64 0 Attributes: AREA_OR_POINT: Area PROCESSING_SOFTWARE: 0.24.0a1 {TIFFTAG_IMAGEDESCRIPTION}: SnowCoveredArea_0=nosnow_1=snow_2-nodatavalu... STATISTICS_MAXIMUM: 1 STATISTICS_MEAN: 1 STATISTICS_MINIMUM: 1 STATISTICS_STDDEV: 0 STATISTICS_VALID_PERCENT: 39.04 _FillValue: 2 scale_factor: 1.0 add_offset: 0.0
Now, we check if the nodata value can be determined directly from the COG metadata
snowmap.rio.nodata
2
Now, we make a plot of the snowmap keeping in mind that 0 = no snow
, 1 = snow
, and 2 = clouds (nodata value)
snowmap.plot(levels=[0, 1, 2])
plt.title("Spatial distribution of snow, no snow and cloudy pixels")
plt.ylabel("Latitude")
plt.xlabel("Longitude")
plt.tight_layout()
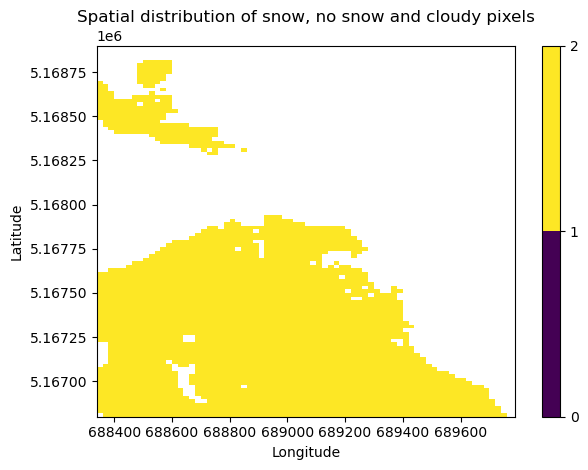
Let’s have a look at the histogram to understand the distribution of the values in our map
data = snowmap.values.flatten()
snowmap.plot.hist(xticks = [0, 1, 2], weights=np.ones(len(data)) / len(data))
plt.gca().yaxis.set_major_formatter(PercentFormatter(1))
plt.title("Distribution of snow, no snow and cloud pixels")
plt.show()
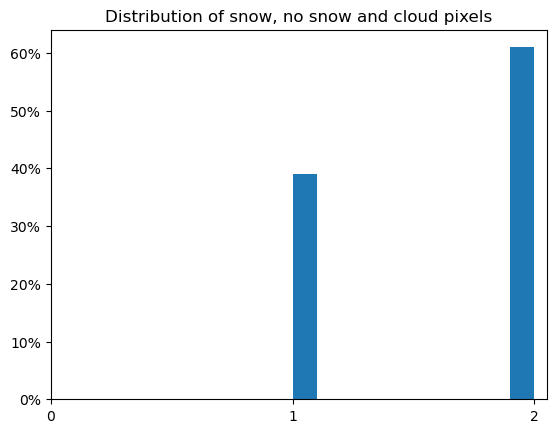
Load STAC metadata#
In addition to the COG we also receive STAC metadata for our result. Let’s have a look at it.
stac_collection = results.get_metadata()
stac_collection
{'assets': {'openEO.tif': {'file:nodata': ['nan'],
'href': 'https://openeo.dataspace.copernicus.eu/openeo/1.2/jobs/j-2401318f19a84122b62657ed798f0c9e/results/assets/MDYxODNjYmMtODRjOC00YzZhLThhN2QtY2IxOGJhMDhjYzhj/ed41e358ad1fe308f681e33015b06869/openEO.tif?expires=1707311403',
'proj:bbox': [688340.0, 5166800.0, 689790.0, 5168900.0],
'proj:epsg': 32632,
'proj:shape': [145, 210],
'raster:bands': [{'name': '1',
'statistics': {'maximum': 2.0,
'mean': 1.5691625615764,
'minimum': 0.5,
'stddev': 0.47448333824272,
'valid_percent': 100.0}}],
'roles': ['data'],
'title': 'openEO.tif',
'type': 'image/tiff; application=geotiff'}},
'description': 'Results for batch job j-2401318f19a84122b62657ed798f0c9e',
'extent': {'spatial': {'bbox': [[11.461193783940812,
46.62835651400798,
11.479180216059186,
46.64670948599201]]},
'temporal': {'interval': [['2023-02-01T00:00:00Z',
'2023-06-01T00:00:00Z']]}},
'id': 'j-2401318f19a84122b62657ed798f0c9e',
'license': 'proprietary',
'links': [{'href': '/eodata/Sentinel-2/MSI/L2A/2023/02/02/S2A_MSIL2A_20230202T101231_N0509_R022_T32TPS_20230202T142000.SAFE',
'rel': 'derived_from',
'title': 'Derived from /eodata/Sentinel-2/MSI/L2A/2023/02/02/S2A_MSIL2A_20230202T101231_N0509_R022_T32TPS_20230202T142000.SAFE',
'type': 'application/json'},
{'href': '/eodata/Sentinel-2/MSI/L2A/2023/02/05/S2A_MSIL2A_20230205T102221_N0509_R065_T32TPS_20230205T135958.SAFE',
'rel': 'derived_from',
'title': 'Derived from /eodata/Sentinel-2/MSI/L2A/2023/02/05/S2A_MSIL2A_20230205T102221_N0509_R065_T32TPS_20230205T135958.SAFE',
'type': 'application/json'},
{'href': '/eodata/Sentinel-2/MSI/L2A/2023/02/07/S2B_MSIL2A_20230207T101109_N0509_R022_T32TPS_20230207T124736.SAFE',
'rel': 'derived_from',
'title': 'Derived from /eodata/Sentinel-2/MSI/L2A/2023/02/07/S2B_MSIL2A_20230207T101109_N0509_R022_T32TPS_20230207T124736.SAFE',
'type': 'application/json'},
{'href': '/eodata/Sentinel-2/MSI/L2A/2023/02/10/S2B_MSIL2A_20230210T102049_N0509_R065_T32TPS_20230210T125716.SAFE',
'rel': 'derived_from',
'title': 'Derived from /eodata/Sentinel-2/MSI/L2A/2023/02/10/S2B_MSIL2A_20230210T102049_N0509_R065_T32TPS_20230210T125716.SAFE',
'type': 'application/json'},
{'href': '/eodata/Sentinel-2/MSI/L2A/2023/02/12/S2A_MSIL2A_20230212T101131_N0509_R022_T32TPS_20230212T142159.SAFE',
'rel': 'derived_from',
'title': 'Derived from /eodata/Sentinel-2/MSI/L2A/2023/02/12/S2A_MSIL2A_20230212T101131_N0509_R022_T32TPS_20230212T142159.SAFE',
'type': 'application/json'},
{'href': '/eodata/Sentinel-2/MSI/L2A/2023/02/15/S2A_MSIL2A_20230215T102121_N0509_R065_T32TPS_20230215T141008.SAFE',
'rel': 'derived_from',
'title': 'Derived from /eodata/Sentinel-2/MSI/L2A/2023/02/15/S2A_MSIL2A_20230215T102121_N0509_R065_T32TPS_20230215T141008.SAFE',
'type': 'application/json'},
{'href': '/eodata/Sentinel-2/MSI/L2A/2023/02/17/S2B_MSIL2A_20230217T101029_N0509_R022_T32TPS_20230217T125054.SAFE',
'rel': 'derived_from',
'title': 'Derived from /eodata/Sentinel-2/MSI/L2A/2023/02/17/S2B_MSIL2A_20230217T101029_N0509_R022_T32TPS_20230217T125054.SAFE',
'type': 'application/json'},
{'href': '/eodata/Sentinel-2/MSI/L2A/2023/02/20/S2B_MSIL2A_20230220T101949_N0509_R065_T32TPS_20230220T145403.SAFE',
'rel': 'derived_from',
'title': 'Derived from /eodata/Sentinel-2/MSI/L2A/2023/02/20/S2B_MSIL2A_20230220T101949_N0509_R065_T32TPS_20230220T145403.SAFE',
'type': 'application/json'},
{'href': '/eodata/Sentinel-2/MSI/L2A/2023/02/22/S2A_MSIL2A_20230222T101031_N0509_R022_T32TPS_20230222T141055.SAFE',
'rel': 'derived_from',
'title': 'Derived from /eodata/Sentinel-2/MSI/L2A/2023/02/22/S2A_MSIL2A_20230222T101031_N0509_R022_T32TPS_20230222T141055.SAFE',
'type': 'application/json'},
{'href': '/eodata/Sentinel-2/MSI/L2A/2023/02/25/S2A_MSIL2A_20230225T102021_N0509_R065_T32TPS_20230225T163245.SAFE',
'rel': 'derived_from',
'title': 'Derived from /eodata/Sentinel-2/MSI/L2A/2023/02/25/S2A_MSIL2A_20230225T102021_N0509_R065_T32TPS_20230225T163245.SAFE',
'type': 'application/json'},
{'href': '/eodata/Sentinel-2/MSI/L2A/2023/02/27/S2B_MSIL2A_20230227T101029_N0509_R022_T32TPS_20230227T124853.SAFE',
'rel': 'derived_from',
'title': 'Derived from /eodata/Sentinel-2/MSI/L2A/2023/02/27/S2B_MSIL2A_20230227T101029_N0509_R022_T32TPS_20230227T124853.SAFE',
'type': 'application/json'},
{'href': '/eodata/Sentinel-2/MSI/L2A/2023/03/02/S2B_MSIL2A_20230302T101839_N0509_R065_T32TPS_20230302T165059.SAFE',
'rel': 'derived_from',
'title': 'Derived from /eodata/Sentinel-2/MSI/L2A/2023/03/02/S2B_MSIL2A_20230302T101839_N0509_R065_T32TPS_20230302T165059.SAFE',
'type': 'application/json'},
{'href': '/eodata/Sentinel-2/MSI/L2A/2023/03/04/S2A_MSIL2A_20230304T101021_N0509_R022_T32TPS_20230907T154000.SAFE',
'rel': 'derived_from',
'title': 'Derived from /eodata/Sentinel-2/MSI/L2A/2023/03/04/S2A_MSIL2A_20230304T101021_N0509_R022_T32TPS_20230907T154000.SAFE',
'type': 'application/json'},
{'href': '/eodata/Sentinel-2/MSI/L2A/2023/03/07/S2A_MSIL2A_20230307T101901_N0509_R065_T32TPS_20230307T162402.SAFE',
'rel': 'derived_from',
'title': 'Derived from /eodata/Sentinel-2/MSI/L2A/2023/03/07/S2A_MSIL2A_20230307T101901_N0509_R065_T32TPS_20230307T162402.SAFE',
'type': 'application/json'},
{'href': '/eodata/Sentinel-2/MSI/L2A/2023/03/09/S2B_MSIL2A_20230309T100749_N0509_R022_T32TPS_20230309T162710.SAFE',
'rel': 'derived_from',
'title': 'Derived from /eodata/Sentinel-2/MSI/L2A/2023/03/09/S2B_MSIL2A_20230309T100749_N0509_R022_T32TPS_20230309T162710.SAFE',
'type': 'application/json'},
{'href': '/eodata/Sentinel-2/MSI/L2A/2023/03/12/S2B_MSIL2A_20230312T101729_N0509_R065_T32TPS_20230312T163807.SAFE',
'rel': 'derived_from',
'title': 'Derived from /eodata/Sentinel-2/MSI/L2A/2023/03/12/S2B_MSIL2A_20230312T101729_N0509_R065_T32TPS_20230312T163807.SAFE',
'type': 'application/json'},
{'href': '/eodata/Sentinel-2/MSI/L2A/2023/03/14/S2A_MSIL2A_20230314T101021_N0509_R022_T32TPS_20230314T161800.SAFE',
'rel': 'derived_from',
'title': 'Derived from /eodata/Sentinel-2/MSI/L2A/2023/03/14/S2A_MSIL2A_20230314T101021_N0509_R022_T32TPS_20230314T161800.SAFE',
'type': 'application/json'},
{'href': '/eodata/Sentinel-2/MSI/L2A/2023/03/17/S2A_MSIL2A_20230317T101741_N0509_R065_T32TPS_20230317T162557.SAFE',
'rel': 'derived_from',
'title': 'Derived from /eodata/Sentinel-2/MSI/L2A/2023/03/17/S2A_MSIL2A_20230317T101741_N0509_R065_T32TPS_20230317T162557.SAFE',
'type': 'application/json'},
{'href': '/eodata/Sentinel-2/MSI/L2A/2023/03/19/S2B_MSIL2A_20230319T100649_N0509_R022_T32TPS_20230319T130804.SAFE',
'rel': 'derived_from',
'title': 'Derived from /eodata/Sentinel-2/MSI/L2A/2023/03/19/S2B_MSIL2A_20230319T100649_N0509_R022_T32TPS_20230319T130804.SAFE',
'type': 'application/json'},
{'href': '/eodata/Sentinel-2/MSI/L2A/2023/03/22/S2B_MSIL2A_20230322T101649_N0509_R065_T32TPS_20230322T145625.SAFE',
'rel': 'derived_from',
'title': 'Derived from /eodata/Sentinel-2/MSI/L2A/2023/03/22/S2B_MSIL2A_20230322T101649_N0509_R065_T32TPS_20230322T145625.SAFE',
'type': 'application/json'},
{'href': '/eodata/Sentinel-2/MSI/L2A/2023/03/24/S2A_MSIL2A_20230324T101021_N0509_R022_T32TPS_20230324T161752.SAFE',
'rel': 'derived_from',
'title': 'Derived from /eodata/Sentinel-2/MSI/L2A/2023/03/24/S2A_MSIL2A_20230324T101021_N0509_R022_T32TPS_20230324T161752.SAFE',
'type': 'application/json'},
{'href': '/eodata/Sentinel-2/MSI/L2A/2023/03/27/S2A_MSIL2A_20230327T101631_N0509_R065_T32TPS_20230327T162303.SAFE',
'rel': 'derived_from',
'title': 'Derived from /eodata/Sentinel-2/MSI/L2A/2023/03/27/S2A_MSIL2A_20230327T101631_N0509_R065_T32TPS_20230327T162303.SAFE',
'type': 'application/json'},
{'href': '/eodata/Sentinel-2/MSI/L2A/2023/03/29/S2B_MSIL2A_20230329T100629_N0509_R022_T32TPS_20230329T130657.SAFE',
'rel': 'derived_from',
'title': 'Derived from /eodata/Sentinel-2/MSI/L2A/2023/03/29/S2B_MSIL2A_20230329T100629_N0509_R022_T32TPS_20230329T130657.SAFE',
'type': 'application/json'},
{'href': '/eodata/Sentinel-2/MSI/L2A/2023/04/01/S2B_MSIL2A_20230401T101559_N0509_R065_T32TPS_20230401T145632.SAFE',
'rel': 'derived_from',
'title': 'Derived from /eodata/Sentinel-2/MSI/L2A/2023/04/01/S2B_MSIL2A_20230401T101559_N0509_R065_T32TPS_20230401T145632.SAFE',
'type': 'application/json'},
{'href': '/eodata/Sentinel-2/MSI/L2A/2023/04/03/S2A_MSIL2A_20230403T100551_N0509_R022_T32TPS_20230403T162459.SAFE',
'rel': 'derived_from',
'title': 'Derived from /eodata/Sentinel-2/MSI/L2A/2023/04/03/S2A_MSIL2A_20230403T100551_N0509_R022_T32TPS_20230403T162459.SAFE',
'type': 'application/json'},
{'href': '/eodata/Sentinel-2/MSI/L2A/2023/04/06/S2A_MSIL2A_20230406T102021_N0509_R065_T32TPS_20230406T194357.SAFE',
'rel': 'derived_from',
'title': 'Derived from /eodata/Sentinel-2/MSI/L2A/2023/04/06/S2A_MSIL2A_20230406T102021_N0509_R065_T32TPS_20230406T194357.SAFE',
'type': 'application/json'},
{'href': '/eodata/Sentinel-2/MSI/L2A/2023/04/08/S2B_MSIL2A_20230408T100559_N0509_R022_T32TPS_20230408T131134.SAFE',
'rel': 'derived_from',
'title': 'Derived from /eodata/Sentinel-2/MSI/L2A/2023/04/08/S2B_MSIL2A_20230408T100559_N0509_R022_T32TPS_20230408T131134.SAFE',
'type': 'application/json'},
{'href': '/eodata/Sentinel-2/MSI/L2A/2023/04/11/S2B_MSIL2A_20230411T101559_N0509_R065_T32TPS_20230411T131019.SAFE',
'rel': 'derived_from',
'title': 'Derived from /eodata/Sentinel-2/MSI/L2A/2023/04/11/S2B_MSIL2A_20230411T101559_N0509_R065_T32TPS_20230411T131019.SAFE',
'type': 'application/json'},
{'href': '/eodata/Sentinel-2/MSI/L2A/2023/04/13/S2A_MSIL2A_20230413T100551_N0509_R022_T32TPS_20230413T161903.SAFE',
'rel': 'derived_from',
'title': 'Derived from /eodata/Sentinel-2/MSI/L2A/2023/04/13/S2A_MSIL2A_20230413T100551_N0509_R022_T32TPS_20230413T161903.SAFE',
'type': 'application/json'},
{'href': '/eodata/Sentinel-2/MSI/L2A/2023/04/16/S2A_MSIL2A_20230416T101601_N0509_R065_T32TPS_20230416T162901.SAFE',
'rel': 'derived_from',
'title': 'Derived from /eodata/Sentinel-2/MSI/L2A/2023/04/16/S2A_MSIL2A_20230416T101601_N0509_R065_T32TPS_20230416T162901.SAFE',
'type': 'application/json'},
{'href': '/eodata/Sentinel-2/MSI/L2A/2023/04/18/S2B_MSIL2A_20230418T100559_N0509_R022_T32TPS_20230418T131202.SAFE',
'rel': 'derived_from',
'title': 'Derived from /eodata/Sentinel-2/MSI/L2A/2023/04/18/S2B_MSIL2A_20230418T100559_N0509_R022_T32TPS_20230418T131202.SAFE',
'type': 'application/json'},
{'href': '/eodata/Sentinel-2/MSI/L2A/2023/04/21/S2B_MSIL2A_20230421T101559_N0509_R065_T32TPS_20230421T131333.SAFE',
'rel': 'derived_from',
'title': 'Derived from /eodata/Sentinel-2/MSI/L2A/2023/04/21/S2B_MSIL2A_20230421T101559_N0509_R065_T32TPS_20230421T131333.SAFE',
'type': 'application/json'},
{'href': '/eodata/Sentinel-2/MSI/L2A/2023/04/23/S2A_MSIL2A_20230423T100551_N0509_R022_T32TPS_20230423T162900.SAFE',
'rel': 'derived_from',
'title': 'Derived from /eodata/Sentinel-2/MSI/L2A/2023/04/23/S2A_MSIL2A_20230423T100551_N0509_R022_T32TPS_20230423T162900.SAFE',
'type': 'application/json'},
{'href': '/eodata/Sentinel-2/MSI/L2A/2023/04/26/S2A_MSIL2A_20230426T101601_N0509_R065_T32TPS_20230426T162055.SAFE',
'rel': 'derived_from',
'title': 'Derived from /eodata/Sentinel-2/MSI/L2A/2023/04/26/S2A_MSIL2A_20230426T101601_N0509_R065_T32TPS_20230426T162055.SAFE',
'type': 'application/json'},
{'href': '/eodata/Sentinel-2/MSI/L2A/2023/04/28/S2B_MSIL2A_20230428T100559_N0509_R022_T32TPS_20230428T133214.SAFE',
'rel': 'derived_from',
'title': 'Derived from /eodata/Sentinel-2/MSI/L2A/2023/04/28/S2B_MSIL2A_20230428T100559_N0509_R022_T32TPS_20230428T133214.SAFE',
'type': 'application/json'},
{'href': '/eodata/Sentinel-2/MSI/L2A/2023/05/01/S2B_MSIL2A_20230501T101559_N0509_R065_T32TPS_20230501T132325.SAFE',
'rel': 'derived_from',
'title': 'Derived from /eodata/Sentinel-2/MSI/L2A/2023/05/01/S2B_MSIL2A_20230501T101559_N0509_R065_T32TPS_20230501T132325.SAFE',
'type': 'application/json'},
{'href': '/eodata/Sentinel-2/MSI/L2A/2023/05/03/S2A_MSIL2A_20230503T101021_N0509_R022_T32TPS_20230503T180406.SAFE',
'rel': 'derived_from',
'title': 'Derived from /eodata/Sentinel-2/MSI/L2A/2023/05/03/S2A_MSIL2A_20230503T101021_N0509_R022_T32TPS_20230503T180406.SAFE',
'type': 'application/json'},
{'href': '/eodata/Sentinel-2/MSI/L2A/2023/05/06/S2A_MSIL2A_20230506T101601_N0509_R065_T32TPS_20230506T162659.SAFE',
'rel': 'derived_from',
'title': 'Derived from /eodata/Sentinel-2/MSI/L2A/2023/05/06/S2A_MSIL2A_20230506T101601_N0509_R065_T32TPS_20230506T162659.SAFE',
'type': 'application/json'},
{'href': '/eodata/Sentinel-2/MSI/L2A/2023/05/08/S2B_MSIL2A_20230508T100559_N0509_R022_T32TPS_20230508T131238.SAFE',
'rel': 'derived_from',
'title': 'Derived from /eodata/Sentinel-2/MSI/L2A/2023/05/08/S2B_MSIL2A_20230508T100559_N0509_R022_T32TPS_20230508T131238.SAFE',
'type': 'application/json'},
{'href': '/eodata/Sentinel-2/MSI/L2A/2023/05/11/S2B_MSIL2A_20230511T101559_N0509_R065_T32TPS_20230511T163715.SAFE',
'rel': 'derived_from',
'title': 'Derived from /eodata/Sentinel-2/MSI/L2A/2023/05/11/S2B_MSIL2A_20230511T101559_N0509_R065_T32TPS_20230511T163715.SAFE',
'type': 'application/json'},
{'href': '/eodata/Sentinel-2/MSI/L2A/2023/05/13/S2A_MSIL2A_20230513T100551_N0509_R022_T32TPS_20230513T161552.SAFE',
'rel': 'derived_from',
'title': 'Derived from /eodata/Sentinel-2/MSI/L2A/2023/05/13/S2A_MSIL2A_20230513T100551_N0509_R022_T32TPS_20230513T161552.SAFE',
'type': 'application/json'},
{'href': '/eodata/Sentinel-2/MSI/L2A/2023/05/16/S2A_MSIL2A_20230516T101601_N0509_R065_T32TPS_20230516T181153.SAFE',
'rel': 'derived_from',
'title': 'Derived from /eodata/Sentinel-2/MSI/L2A/2023/05/16/S2A_MSIL2A_20230516T101601_N0509_R065_T32TPS_20230516T181153.SAFE',
'type': 'application/json'},
{'href': '/eodata/Sentinel-2/MSI/L2A/2023/05/18/S2B_MSIL2A_20230518T100559_N0509_R022_T32TPS_20230518T144255.SAFE',
'rel': 'derived_from',
'title': 'Derived from /eodata/Sentinel-2/MSI/L2A/2023/05/18/S2B_MSIL2A_20230518T100559_N0509_R022_T32TPS_20230518T144255.SAFE',
'type': 'application/json'},
{'href': '/eodata/Sentinel-2/MSI/L2A/2023/05/21/S2B_MSIL2A_20230521T101609_N0509_R065_T32TPS_20230521T131745.SAFE',
'rel': 'derived_from',
'title': 'Derived from /eodata/Sentinel-2/MSI/L2A/2023/05/21/S2B_MSIL2A_20230521T101609_N0509_R065_T32TPS_20230521T131745.SAFE',
'type': 'application/json'},
{'href': '/eodata/Sentinel-2/MSI/L2A/2023/05/23/S2A_MSIL2A_20230523T100601_N0509_R022_T32TPS_20230523T161057.SAFE',
'rel': 'derived_from',
'title': 'Derived from /eodata/Sentinel-2/MSI/L2A/2023/05/23/S2A_MSIL2A_20230523T100601_N0509_R022_T32TPS_20230523T161057.SAFE',
'type': 'application/json'},
{'href': '/eodata/Sentinel-2/MSI/L2A/2023/05/26/S2A_MSIL2A_20230526T101601_N0509_R065_T32TPS_20230526T162854.SAFE',
'rel': 'derived_from',
'title': 'Derived from /eodata/Sentinel-2/MSI/L2A/2023/05/26/S2A_MSIL2A_20230526T101601_N0509_R065_T32TPS_20230526T162854.SAFE',
'type': 'application/json'},
{'href': '/eodata/Sentinel-2/MSI/L2A/2023/05/28/S2B_MSIL2A_20230528T100559_N0509_R022_T32TPS_20230528T131159.SAFE',
'rel': 'derived_from',
'title': 'Derived from /eodata/Sentinel-2/MSI/L2A/2023/05/28/S2B_MSIL2A_20230528T100559_N0509_R022_T32TPS_20230528T131159.SAFE',
'type': 'application/json'},
{'href': '/eodata/Sentinel-2/MSI/L2A/2023/05/31/S2B_MSIL2A_20230531T101559_N0509_R065_T32TPS_20230531T131936.SAFE',
'rel': 'derived_from',
'title': 'Derived from /eodata/Sentinel-2/MSI/L2A/2023/05/31/S2B_MSIL2A_20230531T101559_N0509_R065_T32TPS_20230531T131936.SAFE',
'type': 'application/json'},
{'href': 'https://openeo.dataspace.copernicus.eu/openeo/1.2/jobs/j-2401318f19a84122b62657ed798f0c9e/results',
'rel': 'self',
'type': 'application/json'},
{'href': 'https://openeo.dataspace.copernicus.eu/openeo/1.2/jobs/j-2401318f19a84122b62657ed798f0c9e/results/MDYxODNjYmMtODRjOC00YzZhLThhN2QtY2IxOGJhMDhjYzhj/e967c4ba33e20f0757876cf974ef7eb7?expires=1707311403',
'rel': 'canonical',
'type': 'application/json'},
{'href': 'http://ceos.org/ard/files/PFS/SR/v5.0/CARD4L_Product_Family_Specification_Surface_Reflectance-v5.0.pdf',
'rel': 'card4l-document',
'type': 'application/pdf'},
{'href': 'https://openeo.dataspace.copernicus.eu/openeo/1.2/jobs/j-2401318f19a84122b62657ed798f0c9e/results/items/MDYxODNjYmMtODRjOC00YzZhLThhN2QtY2IxOGJhMDhjYzhj/ed41e358ad1fe308f681e33015b06869/openEO.tif?expires=1707311403',
'rel': 'item',
'type': 'application/geo+json'}],
'openeo:status': 'finished',
'providers': [{'description': 'This data was processed on an openEO backend maintained by VITO.',
'name': 'VITO',
'processing:expression': [{'expression': {'loadcollection1': {'arguments': {'bands': ['B03',
'B11',
'SCL'],
'id': 'SENTINEL2_L2A',
'spatial_extent': {'crs': 4326,
'east': 11.479180216059186,
'north': 46.64670948599201,
'south': 46.62835651400798,
'west': 11.461193783940812},
'temporal_extent': ['2023-02-01', '2023-06-01']},
'process_id': 'load_collection'},
'mask1': {'arguments': {'data': {'from_node': 'reducedimension1'},
'mask': {'from_node': 'reducedimension2'},
'replacement': 2},
'process_id': 'mask'},
'reducedimension1': {'arguments': {'data': {'from_node': 'loadcollection1'},
'dimension': 'bands',
'reducer': {'process_graph': {'add1': {'arguments': {'x': {'from_node': 'arrayelement1'},
'y': {'from_node': 'arrayelement2'}},
'process_id': 'add'},
'arrayelement1': {'arguments': {'data': {'from_parameter': 'data'},
'index': 0},
'process_id': 'array_element'},
'arrayelement2': {'arguments': {'data': {'from_parameter': 'data'},
'index': 1},
'process_id': 'array_element'},
'divide1': {'arguments': {'x': {'from_node': 'subtract1'},
'y': {'from_node': 'add1'}},
'process_id': 'divide'},
'gt1': {'arguments': {'x': {'from_node': 'divide1'}, 'y': 0.4},
'process_id': 'gt'},
'multiply1': {'arguments': {'x': {'from_node': 'gt1'}, 'y': 1.0},
'process_id': 'multiply',
'result': True},
'subtract1': {'arguments': {'x': {'from_node': 'arrayelement1'},
'y': {'from_node': 'arrayelement2'}},
'process_id': 'subtract'}}}},
'process_id': 'reduce_dimension'},
'reducedimension2': {'arguments': {'data': {'from_node': 'loadcollection1'},
'dimension': 'bands',
'reducer': {'process_graph': {'arrayelement3': {'arguments': {'data': {'from_parameter': 'data'},
'index': 2},
'process_id': 'array_element'},
'eq1': {'arguments': {'x': {'from_node': 'arrayelement3'}, 'y': 8},
'process_id': 'eq'},
'eq2': {'arguments': {'x': {'from_node': 'arrayelement3'}, 'y': 9},
'process_id': 'eq'},
'eq3': {'arguments': {'x': {'from_node': 'arrayelement3'}, 'y': 3},
'process_id': 'eq'},
'multiply2': {'arguments': {'x': {'from_node': 'or2'}, 'y': 1.0},
'process_id': 'multiply',
'result': True},
'or1': {'arguments': {'x': {'from_node': 'eq1'},
'y': {'from_node': 'eq2'}},
'process_id': 'or'},
'or2': {'arguments': {'x': {'from_node': 'or1'},
'y': {'from_node': 'eq3'}},
'process_id': 'or'}}}},
'process_id': 'reduce_dimension'},
'reducedimension3': {'arguments': {'data': {'from_node': 'mask1'},
'dimension': 't',
'reducer': {'process_graph': {'median1': {'arguments': {'data': {'from_parameter': 'data'}},
'process_id': 'median',
'result': True}}}},
'process_id': 'reduce_dimension'},
'saveresult1': {'arguments': {'data': {'from_node': 'reducedimension3'},
'format': 'GTiff',
'options': {}},
'process_id': 'save_result',
'result': True}},
'format': 'openeo'}],
'processing:facility': 'openEO Geotrellis backend',
'processing:software': {'Geotrellis backend': '0.24.0a1'},
'roles': ['processor']}],
'stac_extensions': ['https://stac-extensions.github.io/eo/v1.1.0/schema.json',
'https://stac-extensions.github.io/file/v2.1.0/schema.json',
'https://stac-extensions.github.io/processing/v1.1.0/schema.json',
'https://stac-extensions.github.io/projection/v1.1.0/schema.json'],
'stac_version': '1.0.0',
'summaries': {'instruments': []},
'title': 'snowmap_cog',
'type': 'Collection'}
Now it is time to upload solution to the submission folder and make results visible in STAC browser#
Upload both the STAC json file and the final .tif file to “submissions” folder in your home directory
You can use the code below to copy the results to the submissions folder
!cp ./33_results/stac_item.json ~/submissions/
!cp ./33_results/openEO_uint8.tif ~/submissions/
And now by executing the cell below, update of the STAC browser will start. By this, you are uploading your results to the openly available STAC browser. This might take some minutes.
env_var1 = os.getenv('EMAIL')
curl_command = f"curl -X POST -F token=glptt-42d31ac6f592a9e321d0e4877e654dc50dcf4854 -F ref=main -F 'variables[USER_DIRECTORY]=\"{env_var1}\"' https://gitlab.eox.at/api/v4/projects/554/trigger/pipeline"
process = subprocess.Popen(curl_command, shell=True, stdout=subprocess.PIPE, stderr=subprocess.PIPE)
stdout, stderr = process.communicate()
Your results are online!#
You can now browse your results together with all the other submissions at the publicly available STAC Catalog! You can check your snow cover map, that you are correctly listed as the author and that your contribution has the correct name. The license on the STAC Collection “Cubes and Clouds: Snow Cover” is CC-BY-4.0. The STAC Collection also has it’s own DOI.
Congratulations you have just contributed to a community mapping project that is completely open source, open data and FAIR! Make sure to show it also to your friends, colleagues or potential employers :)
https://esa.pages.eox.at/cubes-and-clouds-catalog/browser/#/?.language=en
If you would like to redo your submission, you can still update your files in submissions folder and once ready, run again the code in the cell above.
Attention: If you have previously opened the STAC browser, your old submission will be cached and not directly displayed. To circumvent this, open a private window from your browser.
Happy coding!